Switch Pro Controller Vibration. And I believe Justin said that when interfacing with the controller via Windows DInput, it reported that the controller did not support rumble. It gives a slight rumble. Does anyon have any ideas on how to maybe create a specialized driver for the. Related: windows 7 network adapter, network adapter windows 7, windows 7 drivers, ethernet controller driver for windows 7 Filter Intel Network Adapter Driver for Windows 7. Windows 10 Audio Driver Change Windows 10 won't let me switch to Realtek audio drivers, it always says that 'The best drivers for your device are already installed' after I point it to the file with the Realtek audio driver.
-->A USB Type-C Connector System Software Interface (UCSI) driver serves as the controller driver for a USB Type-C system with an embedded controller (EC).
If your system that implements Platform Policy Manager (PPM), as described in the UCSI specification, in an EC that is connected to the system over:
An ACPI transport, you do not need to write a driver. Load the Microsoft provided in-box driver, (UcmUcsiCx.sys and UcmUcsiAcpiClient.sys). (See UCSI driver).
A non-ACPI transport, such as USB, PCI, I2C or UART, you need to write a client driver for the controller.
If your USB Type-C hardware does not have the capability of handling the power delivery (PD) state machine, consider writing a USB Type-C port controller driver. For more information, see Write a USB Type-C port controller driver.
Starting in Windows 10, version 1809, a new class extension for UCSI (UcmUcsiCx.sys) has been added,which implements the UCSI specification in a transport agnostic way. With minimal amount of code, your driver, which is a client to UcmUcsiCx, can communicate with the USB Type-C hardware over non-ACPI transport. This topic describes the services provided by the UCSI class extension and the expected behavior of the client driver.
Official specifications
Applies to:
- Windows 10, version 1809
WDF version
- KMDF version 1.27
Important APIs
Sample
Replace the ACPI portions with your implementation for the required bus.
UCSI class extension architecture
UCSI class extension, UcmUcsiCx, allows you to write a driver that communicates with its embedded controller by using non-ACPI transport. The controller driver is a client driver to UcmUcsiCx. UcmUcsiCx is in turn a client to USB connector manager (UCM). Hence, UcmUcsiCx does not make any policy decisions of its own. Instead, it implements policies provided by UCM. UcmUcsiCx implements state machines for handling Platform Policy Manager (PPM) notifications from the client driver and sends commands to implement UCM policy decisions, allowing for a more reliable problem detection and error handling.
OS Policy Manager (OPM)
OS Policy Manager (OPM) implements the logic to interact with PPM, as described in the UCSI specification. OPM is responsible for:
- Converting UCM policies into UCSI commands and UCSI notifications into UCM notifications.
- Sending UCSI commands required for initializing PPM, detecting error, and recovery mechanisms.
Handling UCSI commands
A typical operation involves several commands to be completed by the UCSI-complicant hardware. For example, let's consider the GET_CONNECTOR_STATUS command.
- The PPM firmware sends a connect change notification to the UcmUcsiCx/client driver.
- In response, the UcmUcsiCx/client driver sends a GET_CONNECTOR_STATUS command back to the PPM firmware.
- The PPM firmware executes GET_CONNECTOR_STATUS and asynchronously sends a command-complete notification to the UcmUcsiCx/client driver. That notification contains data about the actual connect status.
- The UcmUcsiCx/client driver processes that status information and sends an ACK_CC_CI to the PPM firmware.
- The PPM firmware executes ACK_CC_CI and asynchronously sends a command-complete notification to the UcmUcsiCx/client driver.
- The UcmUcsiCx/client driver considers the GET_CONNECTOR_STATUS command to be complete.
Controller Drivers For Windows 10
Communication with Platform Policy Manager (PPM)
UcmUcsiCx abstracts the details of sending UCSI commands from OPM to the PPM firmware and receiving notifications from the PPM firmware. It converts PPM commands to WDFREQUEST objects and forwards them to the client driver.
Switch Pro Controller Driver Windows -steam Mac
PPM Notifications
The client driver notifies UcmUcsiCx about PPM notifications from the firmware. The driver provides the UCSI data block containing CCI. UcmUcsiCx forwards notifications to OPM and other components which take appropriate actions based on the data.
IOCTLs to the client driver
UcmUcsiCx sends UCSI commands (through IOCTL requests) to client driver to send to the PPM firmware. The driver is responsible for completing the request after it has sent the UCSI command to the firmware.
Handling power transitions
The client driver is the power policy owner.
If the client driver enters a Dx state because of S0-Idle, WDF brings the driver to D0 when the UcmUcsiCx sends a IOCTL containing a UCSI command to the client driver's power-managed queue. The client driver in S0-Idle should reenter a powered state when there is a PPM notification from the firmware because in S0-Idle, PPM notifications are still enabled.
Before you begin...
Determine the type of driver you need to write depending on whether your hardware or firmware implements PD state machine, and the transport.
For more information, see Developing Windows drivers for USB Type-C connectors.
Install Windows 10 for desktop editions (Home, Pro, Enterprise, and Education).
Install the latest Windows Driver Kit (WDK) on your development computer. The kit has the required header files and libraries for writing the client driver, specifically, you'll need:
- The stub library, (UcmUcsiCxStub.lib). The library translates calls made by the client driver and pass them up to the class extension.
- The header file, Ucmucsicx.h.
- The client driver runs in kernel mode and binds to KMDF 1.27 library.
Familiarize yourself with Windows Driver Foundation (WDF). Recommended reading: Developing Drivers with Windows Driver Foundation, written by Penny Orwick and Guy Smith.
1. Register your client driver with UcmUcsiCx
In your EVT_WDF_DRIVER_DEVICE_ADD implementation,
After you have set the Plug and Play and power management event callback functions (WdfDeviceInitSetPnpPowerEventCallbacks), call UcmUcsiDeviceInitInitialize to initialize the WDFDEVICE_INIT opaque structure. The call associates the client driver with the framework.
After creating the framework device object (WDFDEVICE), call UcmUcsiDeviceInitialize to register the client diver with UcmUcsiCx.
2. Create the PPM object with UcmUcsiCx
In your implementation of EVT_WDF_DEVICE_PREPARE_HARDWARE, after you have recieved the list of raw and translated resources, use the resources to prepare the hardware. For example, if your transport is I2C, read the hardware resources to open a communication channel. Next, create a PPM object. To create the object, you need to set certain configuration options.
Provide a handle to the connector collection on the device.
Create the connector collection by calling UcmUcsiConnectorCollectionCreate.
Enumerate the connectors on the device and add them to the collection by calling UcmUcsiConnectorCollectionAddConnector
Decide whether you want to enable the device controller.
Configure and create the PPM object.
Initialize a UCMUCSI_PPM_CONFIG structure by providing the connector handle you created in step 1.
Set UsbDeviceControllerEnabled member to a boolean value determined in step 2.
Set your event callbacks in WDF_OBJECT_ATTRIBUTES.
Call UcmUcsiPpmCreate by passing all the configured structures.
3. Set up IO queues
UcmUcsiCx sends UCSI commands to client driver to send to the PPM firmware. The commands are sent in form of these IOCTL requests in a WDF queue.
The client driver is responsible for creating and registering that queue to UcmUcsiCx by calling UcmUcsiPpmSetUcsiCommandRequestQueue. The queue must be power-managed.
UcmUcsiCx guarantees that there can be at most one outstanding request in the WDF queue. The client driver also is responsible of completing the WDF request after it has sent the UCSI command to the firmware.
Typically the driver sets up queues in its implementation of EVT_WDF_DEVICE_PREPARE_HARDWARE.
Also, the client driver must also call UcmUcsiPpmStart to notify UcmUcsiCx that the driver is ready to receive the IOCTL requests. We recommend that you make that call in your in EVT_WDF_DEVICE_PREPARE_HARDWARE after creating the WDFQUEUE handle for receiving UCSI commands, through UcmUcsiPpmSetUcsiCommandRequestQueue.Conversely, when the driver does not want to process any more requests, it must call UcmUcsiPpmStop. Do this is in your EVT_WDF_DEVICE_RELEASE_HARDWARE implementation.
4. Handle the IOCTL requests
Consider this example sequence of the events that occurs when a USB Type-C partner is attached to a connector.
- PPM firmware determines an attach event and sends a notification to the client driver.
- Client driver calls UcmUcsiPpmNotification to send that notification to UcmUcsiCx.
- UcmUcsiCx notfies the OPM state machine and it sends a Get Connector Status command to UcmUcsiCx.
- UcmUcsiCx creates a request and sends IOCTL_UCMUCSI_PPM_SEND_UCSI_DATA_BLOCK to the client driver.
- The client driver processes that request and sends the command to the PPM firmware. The driver completes this request asynchronously and sends another notification to UcmUcsiCx.
- On successful command complete notification, the OPM state machine reads the payload (containing connector status info) and notifies UCM of the Type-C attach event.
In this example, the payload also indicated that a change in power delivery negotiation status between the firmware and the port partner was successful. The OPM state machine sends another UCSI command: Get PDOs.Similar to Get Connector Status command, when Get PDOs command completes successfully, the OPM state machine notifies UCM of this event.
The client driver's handler for EVT_WDF_IO_QUEUE_IO_DEVICE_CONTROL is similar to this example code. For information about handling requests, see Request Handlers
- Pros
Comfortable, sturdy one-piece design. $10 less than a pair of Joy-Cons.
- Cons
Expensive compared with most other gamepads.
- Bottom Line
The Switch Pro Controller is a solid, traditional one-piece gamepad for Nintendo's unconventional game system.
Out of the box, the Nintendo Switch is a remarkably functional game system thanks to its Joy-Con controllers. They're a pair of wireless gamepads that can attach to the Switch's sides for portable use, or be held separately when the Switch is docked and connected to a TV or sitting on a table. They also form a good approximation of a conventional gamepad with the included Joy-Con Grip, though they don't feel quite as solid as a one-piece gamepad like the Xbox One Wireless Controller.
- $29.99
- $79.99
- $159.99
- $149.99
- $149.99
- $149.99
- $149.99
Switch Pro Controller Driver Windows -steam Update
For that experience you need to get the optional $69.99 Nintendo Switch Pro Controller. Like the Wii U Pro Controller before it, it provides a satisfyingly familiar gameplay experience that you don't quite get out of box. $70 for a controller is pretty pricey ($10 more than the standard Xbox One or PlayStation 4 wireless controllers), but it's also $10 less than getting another pair of Joy-Cons.
Design
The Pro Controller feels very solid in the hands, comparable with the Xbox One's gamepad in size and just a hair heavier at 8.3 ounces (the Xbox One controller is 8 ounces with two AA batteries). If you tried to identify the two solely by their outline, you'd think they were the same. This means the Pro Controller is pleasingly dense, if not quite as rock-solid as the 12.3-ounce, $150 Xbox Elite Wireless Controller. The Pro Controller is currently only available in black, with matte black grips and a translucent black shell over the controls.
Like the Wii U Pro Controller, the Switch Pro uses an Xbox-style button configuration, with the two analog sticks offset rather than parallel from each other. A digital direction pad sits just below and to the right of the left analog stick, and four face buttons (A/B/X/Y) sit just above and to the right of the right analog stick. Small, round capture, home, plus, and minus buttons sit between them, arranged around a Nintendo Switch logo. The top of the gamepad holds two shoulder buttons on each side (L/ZL and R/ZR), a USB-C port for charging, an indicator LED that shows when the gamepad is plugged in, and a small pairing button. The bottom of the gamepad holds four status LEDs that show it's connected and which player it's set to.
According to Nintendo, the Pro Controller can last up to 40 hours between charges. A five-foot USB-C cable is included, and you can easily plug it into a USB port on the Switch Dock.
More Than Buttons
The Switch Pro Controller is packed with most of the various tricks Nintendo put in each pair of Joy-Cons; the only difference between them is that you can't split the Pro Controller into two halves like the left and right Joy-Cons.
The gamepad features motion controls, HD rumble, and Amiibo functionality (the Switch logo is an NFC zone, and works with both Amiibos and Skylanders figures), like the Joy-Cons. The motion sensors work very well, and I could easily target enemy weak points in The Legend of Zelda: Breath of the Wild. The controller lacks the right Joy-Con's infrared camera, but that feature seems very specifically designed for motion-based games where your hands are separate and not gripping a single controller.
Xinput Switch Pro Controller
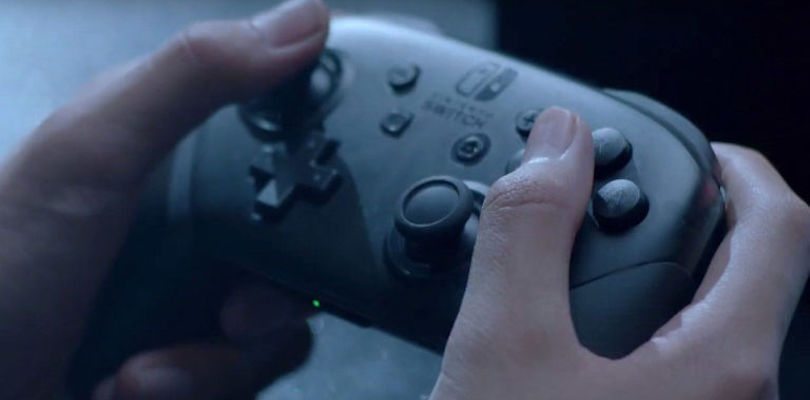
I enjoyed playing Breath of the Wild with the Switch Pro. Its extra heft and solid build felt more substantial than the Joy-Cons in the included grip or the optional Joy-Con Charging Grip. That said, the Joy-Cons feel perfectly fine on their own, and if I didn't have access to the Pro Controller, I don't think I'd particularly miss it. Playing on the Switch with such a beefy gamepad is nice, and you can't do it out of the box, but I don't find it completely necessary.
Should You Go Pro?
Switch Pro Controller Pc Drivers
You don't really need the Nintendo Switch Pro Controller when you have two Joy-Cons and the grip, but it's still an excellent gamepad. It's a conventional controller for an unconventional game system, and it makes games that don't rely on the novelty of the Joy-Cons' modular design feel that much more familiar. If you plan to play more traditional games like Breath of the Wild and Skyrim rather than 1, 2, Switch and Snipperclips, or if the Joy-Cons feel a bit small and light in the grip to you, it's a good investment.
Nintendo Switch Pro Controller
Bottom Line: The Switch Pro Controller is a solid, traditional one-piece gamepad for Nintendo's unconventional game system.